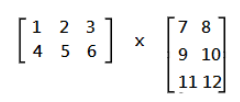
*The number of columns in A need to equal to the number of rows in B.
To calculate that we need to do an action named “dot product”
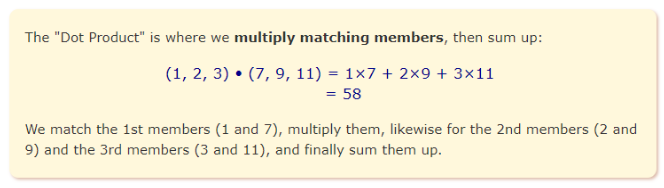
With python, we can use .dot() to calculate the dot product for these 2 matrix:

Example for Multiplying a Matrix by Another Matrix
Question 1)
4*1+5*0 = 4+0 = 4
Result is 4
Python :
import numpy as npResult:
from scipy import linalg
A = np.array([4,5])
B = np.array([[1],[0]])
print(A.dot(B))
[4]
Question 2
From a to f in order, there are the calculation order with use dot product :
a: (1,-2) • (4,-1) = (1*4)+(-2*-1) = 4+2 = 6
b: (0,3) • (4,-1) = (0*4)+(3*-1) = 0-3 = -3
c: (-1,4) • (4,-1) = (-1*4)+(4*-1) = -4-4 = -8
d: (1,3) • (1,2) = (1*4)+(-2*-1) = 1-4 = -3
e: (0,3) • (1,2) = (0*4)+(3*-1) = 0+6 = 6
f: (-1,4) • (1,2) = (-1*4)+(4*-1) = -1+8 = 7
Calculation with Python:
import numpy as npResult :
from scipy import linalg
A = np.array([[1,-2],[0,3],[-1,4]])
B = np.array([[4,1],[-1,2]])
print(A.dot(B))
[4]Reference:
http://chortle.ccsu.edu/vectorlessons/vmch15/vmch15_4.html
Question 3
This is a question from Math is fun website:
https://www.mathsisfun.com/algebra/matrix-multiplying.html
From information, i create 2 matrix:
And the result would be in this format: [a,b,c,d]
a. (3,4,2) • (13,8,6) = (3*13)+(4*8)+(2*6) = 39+32+12 = 83
b. (3,4,2) • (9,7,4) = (3*9)+(4*7)+(2*4) = 27+28+8 = 63
c. (3,4,2) • (7,4,0) = (3*7)+(4*4)+(2*0) = 21+16+0 = 22
d. (3,4,2) • (15,6,3) = (3*15)+(4*6)+(2*3) = 45+24+6 = 75
Result is [$83,$63,$22,$75], which’s means
A. Sold are monday are $83.
B. Sold are Tuesday are $63.
C. Sold are Wedenday are $22.
D. Sold are Thurday are $75.
Calculation with Python:
import numpy as npResult
from scipy import linalg
A = np.array([3,4,2])
B = np.array([[13,9,7,15],[8,7,4,6],[6,4,0,3]])
print(A.dot(B))
[83 63 37 75]
Reference:
http://chortle.ccsu.edu/vectorlessons/vmch15/vmch15_4.html
https://www.mathsisfun.com/algebra/matrix-multiplying.html
No comments :
Post a Comment